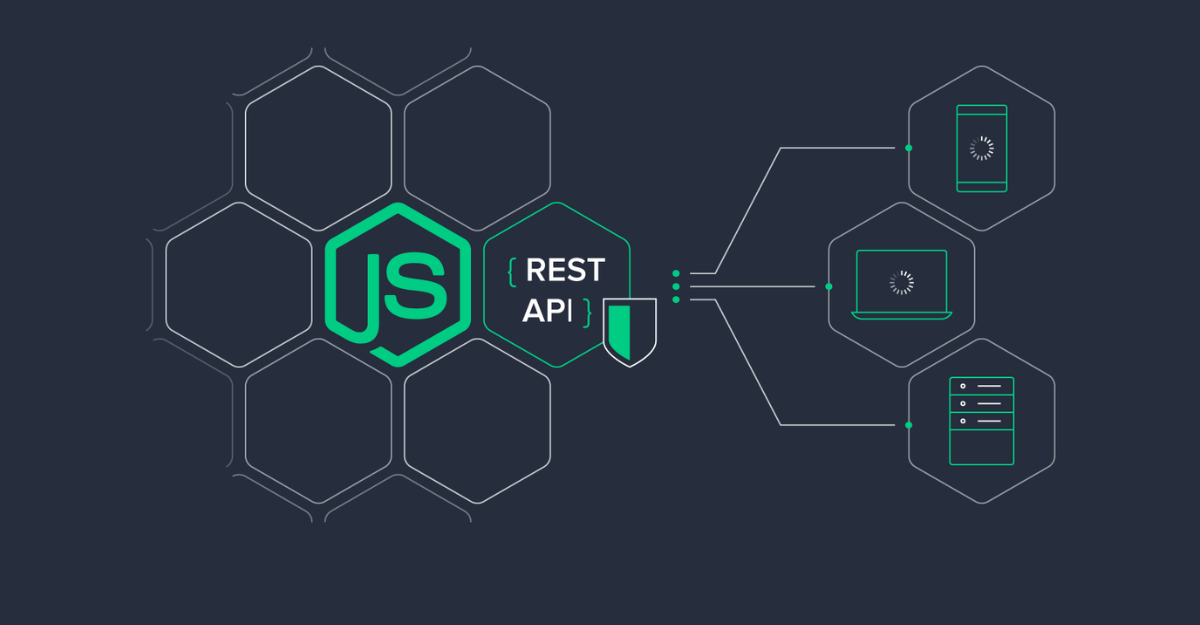
Navigating the World of Node.js Microservices: A Comprehensive Guide
With the digital age, we need better, faster application designs. This led to the Microservices Architecture (MSA). Node.js is essential in MSA with its speedy, event-triggered structure. This post gives a full guide on creating solid microservices using Node.js. It includes top tips, patterns, and real-world examples.
Getting to Know Microservices Architecture in Node.js
Think of MSA as building an app with lots of little services. Each service has its process, talking to others with simple tools. The usual tool is HTTP/REST with JSON. Each service shines alone, but together, they light up the sky. Node.js, using Chrome’s V8 JavaScript engine, is the star player.
In MSA, each service can work separately without waiting on others. This way of working fits Node.js, making it ideal for MSA. It’s like Node.js and MSA were meant to dance together in this digital world.
Imagine a busy kitchen in a top restaurant. Each cook (or service) has their own meal (task) to make. They all must work together smoothly to make a superb meal. The main cook (Node.js), having a full view of all the meals being made, guides the team. This makes sure the kitchen is working smoothly. It watches each cook, making sure they aren’t waiting on others and that they’re using the best parts (modules and tools) they can.
In the Node.js microservices kitchen, there’s zero room for needless waiting or repetition. Each service is made to be as smooth-running as possible. It talks to others only when it needs to and uses resources just right.
Combined, they make a solid, scalable, and highly working app ready to serve many requests from users everywhere. Like our restaurant, the main goal is to give a top user experience. This is only possible with a well-planned Microservices Architecture powered by Node.js. Now that you’ve seen a bit of it, let’s look deeper into why Node.js is a top pick for microservices in our next section.
Why Node.js is a Good Choice for Microservices?
To understand why Node.js often shines in the microservices area, we must look closer at its unique features and strengths. First, Node.js is as light as a feather. Its light development framework pairs well with microservices. These prioritize efficiency and speed. Like a skilled actor, Node.js can do many roles at once. Thanks to its asynchronous, event-driven nature, it can handle many service requests smoothly and without delay.
Node.js stands out with striking features. npm supports it, akin to a toolbox, packed with modules simplifying a developer’s tasks.
It handles multiple requests at once, just like a stage manager effortlessly carrying out many scenes. That’s the magic of Node.js.
Node.js works wonders in managing microservices. Its proficiencies for lean development, handling multiple requests, and marvelous npm support, place Node.js in a commanding position among microservices. Still wondering how to best use these features? Our next section will answer it. Keep reading!
Effective Tips to Build Microservices in Node.js
Crafting sturdy microservices with Node.js calls for specific practices. Much like guidelines for a perfect dance routine, ensuring every move is accurate. We begin with the essential: outlining service borders smoothly. Like dancers adhering to their stage space to prevent mishaps. These borders are defined by business abilities, confirming every service has a unique task.
Firstly, focusing on establishing a strong API gateway is key. Think of it like a traffic controller at an intersection, managing and directing requests to the right microservices. This works as a protective layer, offering a single point of entry for clients and shielding the microservices from direct exposure.
Much like the precision in building a Lego model, code quality is critical. Each piece of code needs to be clearly written and easy to maintain, making the application sturdy and reliable. Regular check-ups on the code, testing, and sticking to top-notch coding rules will help keep the code quality high.
Resilience is just as key. As a boy scout continues his journey even if he stumbles, the system should be built tough enough to isolate any hiccups and recover swiftly. One minor setback shouldn’t snowball to others, affecting the whole operation.
Next, decentralized data management takes the spotlight. Imagine this as giving each microservice its own playground, completely independent to the rest. This makes sure that data stays close to its respective service, strengthening the overall system.
To keep things up-to-date and snappy, the deployment procedure should be automatic. This allows for speedy release of adjustments or repairs, making the operation adaptable and quick-witted.
Abiding by these standard practices will enable you to orchestrate a harmonious array of microservices using Node.js, crafting a resilient, effective, and scalable application.
Using Design Patterns in Node.js Microservices
Within the world of Node.js microservices, design patterns are like magic dance moves. They assist developers in crafting graceful solutions for common issues. These patterns make development simpler, improve code, and increase the efficiency of the microservices system.
Consider design patterns as a dance plan created by a choreographer. Like a choreographer designing steps to suit the music and performance vision, developers use design patterns for their microservices layout.
A typical design pattern in Node.js microservices is the Aggregator Pattern. Imagine a dance captain who brings all the dancers together on stage. In our online dance, this Pattern groups data from multiple services. It makes sure all parts are in line and deliver a seamless user experience.
The Proxy Pattern is another tool in our box. Think of it like the stage manager who controls who goes on stage. A Proxy Pattern, in Node.js microservices, serves as a gatekeeper. It regulates how other services interact with a specific service. It’s essential in making sure the correct service is used when needed, which boosts the application’s efficiency.
Lastly, we have the Chained, or Chain of Responsibility Pattern. Picture a relay race where batons are passed from one runner to another. Each runner, or in this case, service, has a specific task. After fulfilling its task, it hands over control to the next service. This pattern separates the command sender from the receiver. It ensures that services work independently, which boosts the system’s modularity and scalability.
These design patterns in Node.js microservices can be compared to the precise movements of a top-tier ballet performance. Each pattern, like a dancer, adds to the overall rhythm, helping make an effective, speedy, and sturdy application. The difficulty is in picking the correct pattern for each service, like choosing the perfect dance step for the beat. But when you get the hang of it, these patterns can help create a breathtaking show of microservices using Node.js.
Real-World Examples of Successful Node.js Microservices
We’ve touched on the theory. Let’s look at actual examples of companies using Node.js microservices to their advantage. Netflix and LinkedIn are two giants that have seen substantial advantages from this method.
Netflix, a worldwide streaming behemoth, had to serve millions of viewers, each having individual preferences and viewing behaviors. They needed a setup that was nimble, effective, and ensured a seamless user experience. That’s where Node.js microservices came in. Netflix utilized them to boost their app’s performance and user experience. The outcome? Faster load times, less troubleshooting, and a highly responsive UI that thrilled their global audience. Now that’s a standout performance!
In the professional networking world, LinkedIn faced a similar hurdle. They needed to handle an exponentially increasing user base while keeping their mobile app quick and effective. LinkedIn turned to Node.js microservices and saw remarkable improvements. They managed to cut their server number from 30 down to just 3. This structural change vastly enhanced their mobile app’s speed and efficiency, ameliorating the user experience and simplifying professional networking for their millions of users.
Let’s look at how Node.js microservices affect app functions and the user’s experience. Both Netflix and LinkedIn used Node.js microservices. They saw improvements in speed, strength, and flexibility. This helps them serve their large number of users. Other companies can use this as an example when updating their digital platforms!
Facing and Solving Problems when Creating Microservices with Node.js
Creating a microservices setup with Node.js is like putting together a very tricky dance routine. The final product can seem perfect, but there will be problems along the way. But don’t worry, we can turn these problems into advantages to help improve the app. Let’s talk about the common issues to deal with – managing services, communicating between services, and keeping data the same everywhere.
Think of managing services as a dance choreographer ensuring all dancers are right on cue. With services working on their own timing, this can be a tough job. Using a managing process that fits with the needs of your business can help handle this difficulty and ensure smooth operations.
Then, there is the task of making sure all services can talk to each other smoothly. This is like dancers keeping in step together. Here, using modes of communication like REST or gRPC helps keep services chatting and working together without a hitch.
Think of data consistency as a dance routine where everyone needs to know their part. In a system like microservices, where each service has its own database, maintaining consistency can be tough. But with techniques like Event Sourcing or CQRS (Command Query Responsibility Segregation) it becomes manageable. These techniques act as dance instructors, making sure each service maintains its data consistency.
These challenges aren’t easy to beat. But the right tactics and tools can help. They allow you to tackle problems head-on with your Node.js microservices. And even gracefully leap over some issues! Remember, each challenge offers a chance to tweak your strategy and deliver a performance that’s adaptable, efficient, and strong. The trick is to stay focused, keep improving, and never stop learning new moves in this lively dance of Node.js microservices.
Boost developer productivity and reduce costs with our Node.JS development company. Contact us for a project estimation
Conclusion
Mapping out the world of Node.js microservices is like choreographing a complex ballet. Each service executes its role precisely and in sync with others. As discussed in this detailed guide, Node.js stands out as a prime choice for building strong microservices. This is due to its streamlined framework, asynchronous operation, and a rich set of tools.
The emphasis on following best practices makes the performance flawless. These practices range from setting clear service limits to automating deployment, ensuring the performance is pitch-perfect.
We’ve discovered how picking the right design patterns equates to choosing the best dance steps for our Node.js microservices routine. Patterns like the Aggregator or Proxy provide nifty solutions to typical hurdles. This makes for a more productive and sturdy app.
By examining real-world examples like Netflix and LinkedIn, we’ve seen the majesty of a well-implemented Node.js microservices framework. It reminded us that even though this digital dance is complex, with dedication and practice, a spectacular show is achievable.
Lastly, we tackled potential roadblocks in this endeavor. Like a skilled ballet company, we can outmaneuver these with proper strategies and a tough spirit. In fact, every problem faced is a chance to perfect our routine.
In closing, adopting Node.js microservices feels like taking part in a grand digital dance. It might appear intimidating at the onset, but with training, determination, and a handy guide, you can conquer this art. So, cheers to your venture into this thrilling realm of Node.js microservices. May this manual serve as your dance tutor, guiding you towards a mesmerizing, efficient digital display that enchants your spectators – the users. On that cue, let the dance commence!
Frequently Asked Questions
1. What is Node.js?
Node.js, built on Chrome’s V8 JavaScript engine, is a JavaScript runtime known for its event-driven architecture and swift performance that avoids blocking.
2. What is Microservices Architecture (MSA)?
MSA is a design approach where an application is structured as a collection of loosely coupled services, which can be developed, deployed, and scaled independently.
3. Why use Node.js for Microservices?
Node.js is lightweight, supports asynchronous processing, and has a rich ecosystem of packages (npm). These features align with the principles of microservices and ensure efficiency and speed.
4. What are the best practices when using Node.js for Microservices?
Key practices include defining clear service boundaries, implementing a robust API gateway, maintaining high code quality, and promoting decentralized data management, among others.
5. What are some Node.js microservices design patterns?
Some common patterns include the Aggregator Pattern, the Proxy Pattern, and the Chain of Responsibility Pattern. Each pattern provides a solution to a common problem in microservices development.
6. How have companies benefitted from using Node.js for microservices?
Netflix and LinkedIn, for example, have seen improved performance, reduced server counts, and enhanced user experiences after transitioning to Node.js microservices.
7. What challenges might arise in building microservices with Node.js?
Service orchestration, inter-service communication, and maintaining data consistency are common challenges, but can be overcome with robust strategies and tools.
8. Is Node.js equipped to deal with a lot of concurrent service requests?
Indeed, Node.js has the power to tackle simultaneous service requests. This is because it features non-blocking input-output operations.
9. Why does every microservice have a separate database?
This approach encourages data uniformity. Moreover, it securely anchors data to its particular service. This makes the entire system sturdier.
10. What’s the function of an API Gateway in a Node.js microservices setting?
It stands as a middle layer. Its chore is to manage and direct incoming requests towards the right microservices. Additionally, it shields these microservices from being directly exposed.